반응형
자바(JAVA) 실습 - TCP Echo Server/Client - 전문 통신 (fixed length)
Server
1. 전문의 첫 8byte에 전문의 전체 길이
2. 41번째 바이트부터 9byte는 인터페이스 아이디 - 인터페이스 아이디를 기준으로 조건 설정
3. 들어온 메세지를 그대로 client에게 전송
4. 전송 후 대기
Client
1. 전문의 첫 8byte에 전문의 전체 길이
2. 41번째 바이트부터 9byte는 인터페이스 아이디 - 인터페이스 아이디를 기준으로 조건 설정
3. 전문을 Server에 전송
4. 들어온 메세지 표시
5. 메세지 표시 후 종료
Server
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
|
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetSocketAddress;
import java.net.ServerSocket;
import java.net.Socket;
public class TcpServerExample {
public static void main(String[] args) {
ServerSocket serverSocket = null;
try {
serverSocket = new ServerSocket();
serverSocket.bind(new InetSocketAddress("localhost", 9091));
while (true) {
System.out.println("\n[ Waiting ... ] \n");
Socket socket = serverSocket.accept();
InetSocketAddress isa = (InetSocketAddress) socket.getRemoteSocketAddress();
System.out.println("\n[ Accept ... ] \n" + isa.getHostName() + ":" + isa.getPort());
byte[] bytes = null;
String header = null;
String message = null;
InputStream is = socket.getInputStream();
DataInputStream dis = new DataInputStream(is);
byte[] header_bytes = new byte[8];
int readHeader = dis.read(header_bytes);
header = new String(header_bytes, 0, readHeader, "UTF-8");
System.out.println("\n[ Data Length ] \n" + header);
int length = Integer.parseInt(header);
bytes = new byte[length];
int readMessage = is.read(bytes);
// Get Interface ID
byte[] if_id_byte = new byte[9];
System.arraycopy(bytes, 33, if_id_byte, 0, if_id_byte.length);
String if_id_str = new String(if_id_byte, "UTF-8");
System.out.println("\n[ Interface ID ] \n" + if_id_str);
message = new String(bytes, 0, readMessage, "UTF-8");
System.out.println("\n[ Data Received ] \n" + message);
OutputStream os = socket.getOutputStream();
DataOutputStream dos = new DataOutputStream(os);
message = header + message;
bytes = message.getBytes("UTF-8");
dos.write(bytes);
dos.flush();
System.out.println("\n[ Data Send Success ] \n" + message);
dos.close();
os.close();
dis.close();
is.close();
socket.close();
System.out.println("\n[ Socket closed ]\n");
}
} catch (Exception e) {
e.printStackTrace();
}
if (!serverSocket.isClosed()) {
try {
serverSocket.close();
System.out.println("\n[ Socket closed ]\n");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
|
Client
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 | import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; import java.net.InetSocketAddress; import java.net.Socket; public class TcpClientExample { public static void main(String[] args) { Socket socket = null; try { socket = new Socket(); System.out.println("\n[ Request ... ]\n"); socket.connect(new InetSocketAddress("localhost", 9091)); System.out.println("\n[ Success ... ]\n"); byte[] bytes = null; String message = null; OutputStream os = socket.getOutputStream(); message = "00000243@@ae-6y@@ IF0000001 127.0.0.1 D1SS202010201016210551101621000202010201016210551UC AA00000058123 234 345 @@"; bytes = message.getBytes("UTF-8"); os.write(bytes); os.flush(); System.out.println("\n[ Data Send Success ]\n" + message); InputStream is = socket.getInputStream(); bytes = new byte[500]; int readByteCount = is.read(bytes); message = new String(bytes, 0, readByteCount, "UTF-8"); System.out.println("\n[ Data Received ]\n" + message); os.close(); is.close(); socket.close(); System.out.println("\n[ Socket closed ]\n"); } catch (Exception e) { e.printStackTrace(); } if (!socket.isClosed()) { try { socket.close(); System.out.println("\n[ Socket closed ]\n"); } catch (IOException e) { e.printStackTrace(); } } } } |
Server
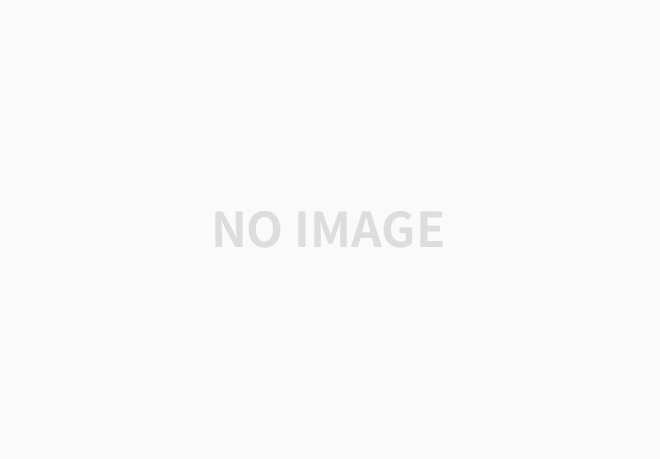
Client
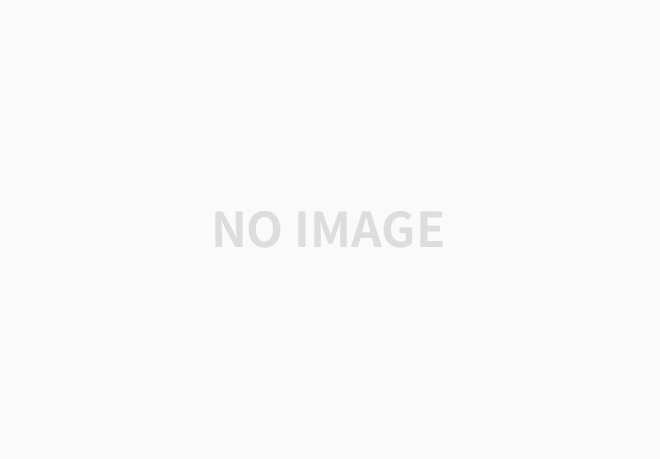
반응형
'JAVA 자바 > JAVA 실습' 카테고리의 다른 글
[JAVA 실습 #5] TCP 전문 통신 (fixed length) - 전문 헤더 읽기 readInt, writeInt 활용 (1) (0) | 2021.02.07 |
---|---|
[JAVA 실습 #4] WAR파일 실행시 IPv4 사용 설정 (0) | 2020.12.20 |
[JAVA 실습 #3] http통신, REST API 호출, json 데이터 처리 - StringBuilder, BufferedReader 활용 (0) | 2020.12.05 |
[JAVA 실습 #3] http통신, REST API 호출, json 데이터 처리 (0) | 2020.11.30 |
[JAVA 실습 #1] 카드번호 마스킹 예시 - StringBuilder, charArray, substring 활용 (0) | 2020.10.17 |